2 min to read
Time Delta - HackerRank Python Solution
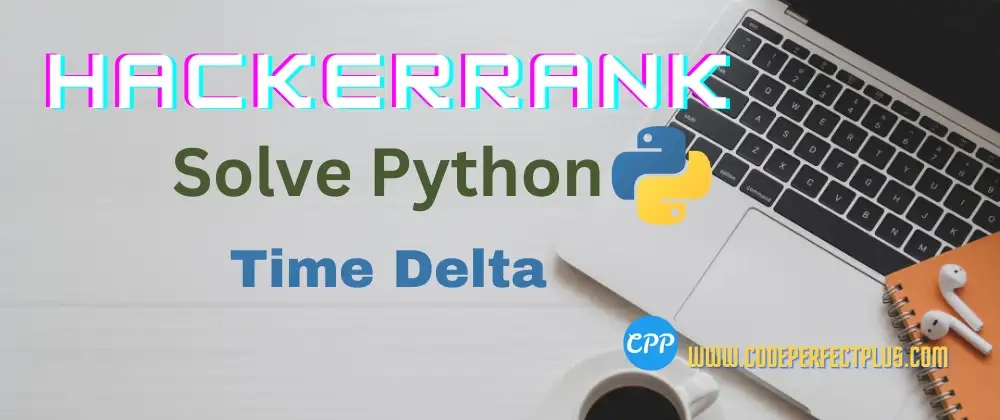
Time Delta is a medium difficulty problem that involves datetime manipulation and we have to return the absolute difference between in seconds. We will learn how to solve this problem in Python through a step-by-step tutorial in Python3.
Problem Statement and Explanation
Given two timestamps in the format Day dd Mon yyyy hh:mm:ss +xxxx
where +xxxx
represents the time zone. We have to print the absolute difference (in seconds) between them.
Suppose the first timestamp is Sun 10 May 2015 13:54:36 -0700
and the second timestamp is Sun 10 May 2015 13:54:36 -0000
.
- t1 = Sun 10 May 2015 13:54:36 -0700
- t2 = Sun 10 May 2015 13:54:36 -0000
The absolute difference between them is 25200 seconds because the time zone offsets are -0700
and -0000
and then 7 hours is 25200 seconds. 7 * 3600 = 25200 seconds.
Time Delta Solution in Python
Explanation of Solution
time_delta()
function takes two strings as input, t1 and t2, which represent two timestamps. The function then converts t1 and t2 to datetime objects using the datetime.datetime.strptime() function.
The datetime.datetime.strptime() function takes a string as its argument and parses it according to a format string. In this case, the format string is %a %d %b %Y %H:%M:%S %z. This format string specifies that the string should be parsed as a date and time in the local time zone.
Once t1 and t2 have been converted to datetime objects, the function then calculates the difference between them using the abs() function and the total_seconds() method. The abs() function returns the absolute value of an expression. The total_seconds() method returns the total number of seconds in a datetime object. It’s important to note that the total_seconds() method returns a float, so we have to convert it to an integer before returning it from the function.
Finally, the function returns the difference between t1 and t2 as a string.
Time Complexity of the Solution
The time complexity of the above solution is O(1)
because we are using the datetime
module to convert the given string into a datetime object and then we are finding the absolute difference between them and returning the difference in seconds.