3 min to read
Plus Minus - HackerRank Problem Solving
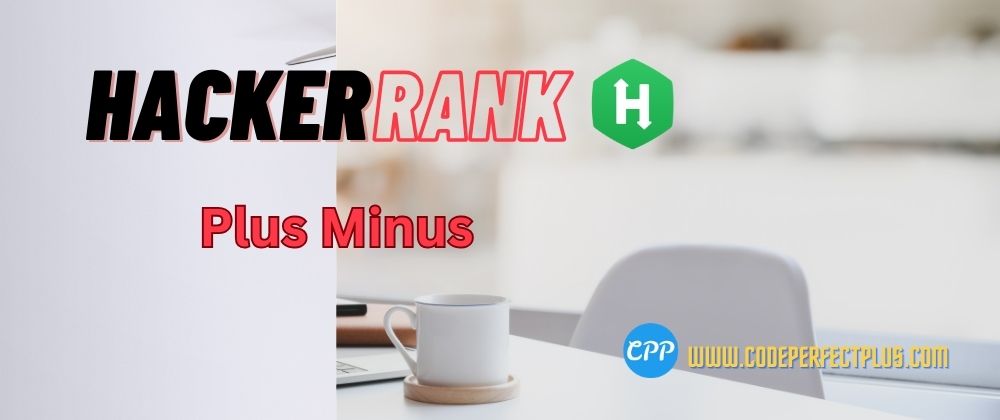
The plus-minus problem is a simple yet challenging programming problem. The goal is to calculate the fraction of positive, negative, and zero numbers in an array. This problem can be solved using a variety of algorithms, including the one presented in this article.
It’s a great exercise for beginners who are looking to hone their programming skills and gain a deeper understanding of Python’s core concepts.
In this post, you will learn how to solve the Plus Minus problem on the Hackerrank website and implement the solution in Python and C++.
Problem Statement and Explanation
In the given array, calculate the ratio of positive, negative and zero. print the decimal value of each fraction on a new line with 6 places after the decimal.
Input Format
The first line contains the n
- number of integers
The second line contains n
space-separated integers describing the array of numbers.
Output Format
-
Print the following 3 lines, each to 6 decimals:
- Ratio of positive numbers
- Ratio of negative numbers
- Ratio of zero numbers
Plus Minus Solution in Python
Plus Minus Solution in C++
Explanation of Solution
The function plusMinus()
takes an array of integers as input and prints the fraction of positive, negative, and zero numbers in the array.
- The function first declares two variables:
countPositive
, andcountNegative
. These variables will be used to store the number of positive and negative in the array, respectively. - The next step is to iterate through the array using a for loop. In each iteration, the code checks if the current element is positive.
- If it is, the
countPositive
variable is incremented by 1. Otherwise, if the current element is negative, thecountNegative
variable is incremented by 1. - Get the total number of
countZero
in the array by subtracting the total number of positive and negative numbers from the total number of elements in the array. - After the loop has finished iterating, the function calculates the fraction of positive, negative, and zero numbers by dividing the corresponding count variable by the total number of elements in the array. -
- Finally, the function prints the three fractions.
Here is an example:
Consider the following array:
arr = [-4, 3, -9, 0, 4, 1]
The function will iterate through the array and count the number of positive, negative, and zero numbers. The result will be as follows:
countPositive = 3
countNegative = 2
countZero = 1
The function will then calculate the fraction of positive, negative, and zero numbers by dividing the corresponding count variable by the total number of elements in the array. The result will be as follows:
positiveFraction = 3/6 = 0.500000
negativeFraction = 2/6 = 0.333333
zeroFraction = 1/6 = 0.166667
Finally, the function will print the three fractions:
Test Case of Plus Minus Problem
Time Complexity of Plus Minus Problem
The time complexity of the above code is O(n), where n is the length of the array. This is because the for loop in the plus_minus() function iterates over the entire array, and each iteration takes a constant amount of time.
Space Complexity of Plus Minus Problem
The space complexity of the plus-minus problem is O(1). This is because the algorithm only uses a constant number of variables, regardless of the size of the input array.
The following are the variables used by the algorithm:
n
: The length of the input array.countPositive
: A counter to keep track of the number of positive numbers in the array.countNegative
: A counter to keep track of the number of negative numbers in the array.countZero
: A counter to keep track of the number of zero numbers in the array.
These variables are all of constant size, so the overall space complexity of the algorithm is O(1).