2 min to read
Mini-Max Sum - HackerRank Problem Solving
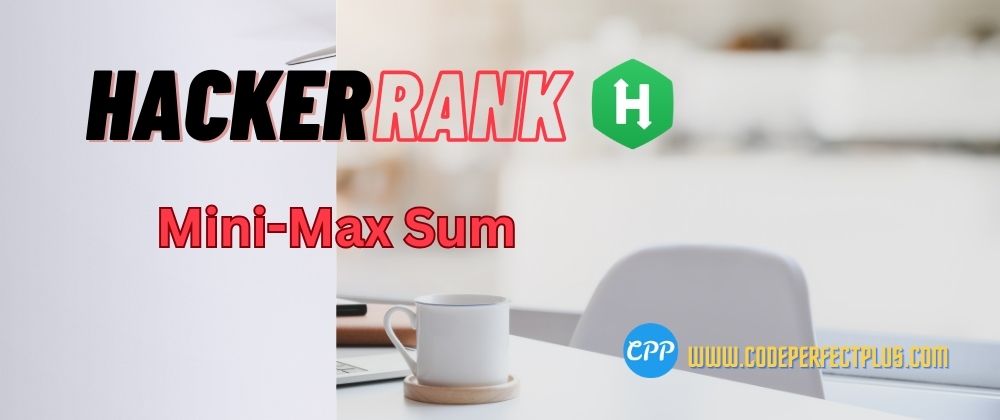
Mini-Max Sum is a Hackerrank problem from Algorithms subdomain that requires the understanding of sum of For-Loop and Array. In this post, you will learn how to solve Hackerrank’s Mini-Max Sum problem and its solution in Python and C++.
Problem Statement and Explanation
Find the maximum and minimum sum of the given array of integers. print the maximum and minimum sum of any four elements of the array.
Example: 2 3 1 4 5
Maximum sum: 3 + 4 + 5 + 2 = 14
Minimum sum: 1 + 2 + 3 + 4 = 10
Mini-Max Sum Solution in Python
Explanation of Solution in Python
The miniMaxSum()
, which takes an array of integers as input and prints the minimum and maximum sum of any four elements of the array.
The function works by first initializing a variable sum
to 0. Then, it iterates over the input array and adds each element to sum
.
After the loop has finished iterating, the function calculates the minimum and maximum sum of any four elements of the array by subtracting the largest and smallest elements from sum
, respectively.
Finally, the function prints the minimum and maximum sum to the console.
Mini-Max Sum Solution in C++
Explanation of Solution in C++
The miniMaxSum()
, which takes a vector of integers as input and prints the minimum and maximum sum of any four elements of the vector.
The function works by first initializing two variables: min
and max
, to the maximum and minimum possible long long values, respectively.
Then, it iterates over the input vector and calculates the sum of all the elements in the vector except for the current element. This is done by using a nested for loop.
For each iteration of the outer loop, the function updates the min and max variables if the calculated sum is less than min or greater than max, respectively.
After the loop has finished iterating, the function prints the values of min and max to the console.