3 min to read
Athlete Sort - HackerRank Solution in Python
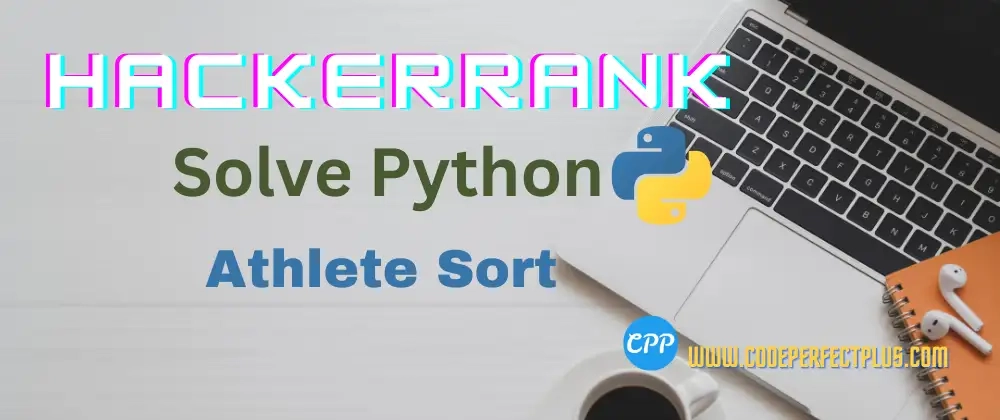
Athlete sort - HackerRank is a medium-difficulty problem of Python based on sorting and nested lists. It is part of the Python practice problems of HackerRank. In this post, we will see how we can solve this challenge in Python
Problem Statement
In this problem statement, we have to sort the given spreadsheet with respect to the Kth
column. The spreadsheet consists of N
rows and M
columns. We have to print the sorted table.
Suppose the given spreadsheet is
Sr. No. | Age | Marks | Height | Weight | Roll No. | class |
---|---|---|---|---|---|---|
1 | 10 | 12 | 170 | 40 | 3 | 8 |
2 | 12 | 12 | 180 | 50 | 1 | 9 |
3 | 8 | 10 | 160 | 45 | 2 | 7 |
and we have to sort the spreadsheet with respect to the Kth
column. Suppose K = 1
then the sorted spreadsheet will be based on the Age
column.
Sr. No. | Age | Marks | Height | Weight | Roll No. | class |
---|---|---|---|---|---|---|
3 | 8 | 10 | 160 | 45 | 2 | 7 |
1 | 10 | 12 | 170 | 40 | 3 | 8 |
2 | 12 | 12 | 180 | 50 | 1 | 9 |
Input Format
- The first line contains
N
andM
separated by a space. - The next
N
lines containM
elements separated by a space. - In the last line, we have to enter the value of
K
.
Output Format
- Print the
N
lines of the sorted table. Each line should contain the space-separated elements.
Constraints
1 <= N, M <= 1000
0 <= K < M
X <= 100
whereX
is any element in the table.
Note: K is indexed from 0
to M-1
i.e. the first column is indexed as 0
.
Sample Output
7 1 0
10 2 5
6 5 9
9 9 9
1 23 12
Athlete Sort HackerRank Solution in Python
Explanation of Solution
The athlete_sort()
the function takes four arguments: the list of athletes arr
, the number of athletes n
, the number of attributes m
, and the kth attribute k
.
The function first sorts the list arr based on the kth attribute using the lambda function. In the lambda function, we are passing the kth attribute as the key to sort the list.
Finally, the function prints the sorted list arr.
The main()
function is responsible for getting the input from the user and calling the athlete_sort() function. The function first gets the number of athletes n and the number of attributes m from the user. Then, it creates an empty list arr to store the athlete data.
The function then reads the athlete data into the list arr. Finally, it calls the athlete_sort()
function to sort the athlete data and print the result.
After sorting the spreadsheet we have to print the sorted spreadsheet. The output of the above spreadsheet will be in the console as
Time Complexity of the Solution
-
The time complexity of the above first solution is
O(n log n)
because we are using the inbuiltsort()
function which has the time complexity ofO(n log n)
. -
The time complexity of the above second solution is
O(n^2)
because we are using the nested loops and comparing the values. If the first value is greater than the second value then we will swap the values.